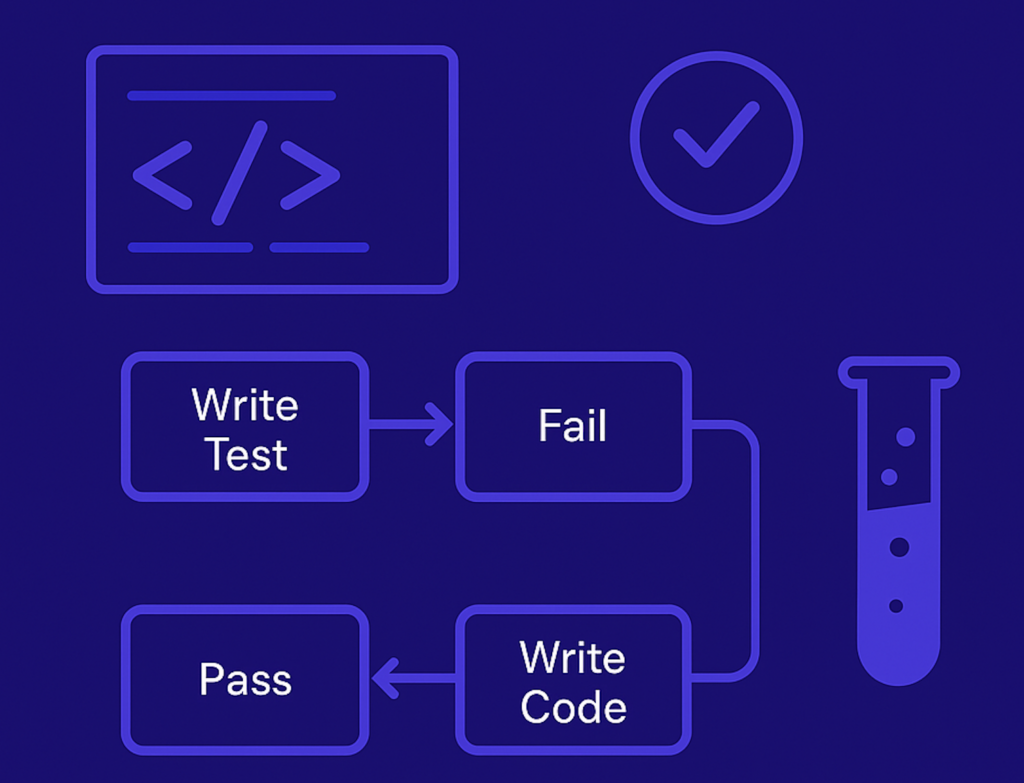
At DPDzero, every backend change must be accompanied by tests. No exceptions. We’ve been doing this since day one — and while many startups believe testing slows down development, we’ve seen the exact opposite.
The Common Myth
There’s a belief that writing tests slows you down and hurts speed, especially in early-stage startups. In reality, not writing tests is what slows you down — especially as your codebase grows and evolves.
The typical development cycle without TDD (Test-Driven Development) looks like this:
- Write backend code
- Write frontend code
- Manually test the flow
- Repeat until it works
This has some major drawbacks:
- You lose context of your backend logic by the time frontend is done
- Your testing is limited to the UI you’ve built
- Fixing one thing often breaks another
- UI-based testing is slow and repetitive, especially when setting up edge cases
Over time, these issues compound. As more features are added, more engineers join, and more bugs surface, shipping confidently becomes harder without a reliable test suite.
Our Philosophy
At DPDzero, good test coverage is a speed enabler. It gives us the confidence to build and ship fast, with fewer bugs and easier maintenance.
Our Development Process
When building new APIs or features:
- Define the API entry point
- Write a few basic test cases:
- Use test utilities to set up required data (e.g., users, dependencies)
- Write 1-2 happy-path tests to validate the core behavior
- Implement the API and ensure those tests pass
- Add more test cases to handle edge cases, failures, and validations
- Refactor the implementation if needed
When modifying existing code:
- For new functionality: Add test cases to validate the new behavior
- For bug fixes: Add test cases that reproduce the bug and confirm the fix
Our Tooling & Enforcement
- CI on every PR: Every pull request runs the full test suite via GitHub Actions. This typically takes around 4 minutes and acts as a gate for merging. If tests fail, the PR can’t be merged.
- Test coverage tracking via SonarQube: We monitor code coverage and quality metrics using SonarQube. It provides visibility into how much of the new or modified code is tested — and flags areas that may need better coverage.
- Manual + Code Review Checks: During code reviews, we always check if the PR includes meaningful test cases. It’s not just about lines of test code — we ask:
- Are key paths covered?
- Are edge cases handled?
- Will this catch regressions?
Our Approach to Mocking
At DPDzero, we follow a “mock only what’s necessary” philosophy when writing tests.
We do not mock core infrastructure components like:
- Databases
- Caches (e.g., Redis)
- Internal services or APIs that are part of the same codebase
Mocking these can lead to misleading tests that don’t reflect real-world behavior. Instead, we rely on integration-style tests that hit actual services (or lightweight local containers) to validate correctness end-to-end.
However, we do mock things that:
- Are external to our system (e.g., third-party APIs)
- Are asynchronous or side-effect driven (e.g., job queues, notification services, webhook triggers)
- Would slow down or complicate tests without adding value to correctness
This approach ensures that our tests are both reliable and realistic. We optimize for fast feedback without sacrificing test fidelity. If your test passes, it’s highly likely the actual feature will work as expected in production.
The Benefits
✅ Instant gratification
Seeing tests turn from red to green is deeply satisfying. It’s a visible sign of progress — and correctness.
⚡ Speed
Writing tests helps you move faster. You verify the backend is solid before wiring up the frontend. Refactors become safe and fast.
🛠 Easy maintenance
Tests stick around. As code changes, tests catch regressions instantly. This keeps the codebase stable even as it evolves.
🧠 Better design and clarity
TDD forces you to think about the API and its usage before implementation. This leads to cleaner, more modular, and well-thought-out code with better separation of concerns.
📉 Fewer bugs in production
By covering edge cases and known failure scenarios early, TDD drastically reduces the likelihood of bugs slipping through to production — especially regressions.
👥 Easier collaboration
Tests act as living documentation. When other developers touch your code later, the tests tell them how it’s supposed to behave. This makes collaboration smoother and onboarding faster.
🔄 Confidence to refactor
When you want to improve or clean up code, having a strong test suite gives you the confidence to make changes without fear of unintended breakage.
Writing tests doesn’t slow us down — it’s how we move fast and break nothing. That’s why we’re proud to be dogmatic about testing at DPDzero.